The grammar is strict because of its unique philosophy. Apart from whether it works well, there is even a style guide that recommends writing like this for readability. Python official documents recommend keeping the coding style suitable for Python, which is PEP 8.
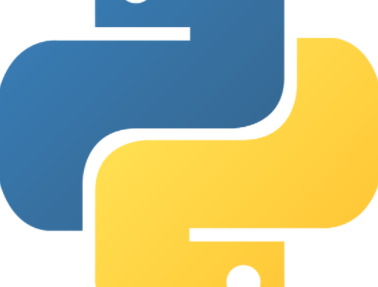
In other languages, brackets are usually used in block units, but in Python, indentation is used instead of brackets. Because of this indentation grammar, the official coding guide specified in PEP 8 strongly recommends putting four characters of Space instead of Tab characters in source code indentation. Tab characters have different widths of spaces according to settings of a user or system. The problem is that the Python interpreter processes one tap character as one blank character. If you process the indentation with a tap character, the code indentation will be broken with a high probability if you open it in an editor with a different tap setting, and the source code will be broken in a form that humans cannot fix (visual block but Python interpreter recognizes as another block). Of course, programmers cannot let go of the annoying act of hitting the space bar four times. The latest editors used by Python developers, such as PyCharm and VSCode, support the ability to automatically convert into four gaps and input them, so using these editors eliminates the need to bother four gaps.
In Python, no matter how you name a variable or class, it works well. However, PEP 8 also specifies the recommended style for this. When coding Python, it is recommended to follow this recommended style for readability. Below is a representative style guide for names.
MODULE_CONSTANT_NAME = 0
import module_name
class ClassName()
def function_name()
variable_name = 0
_protected_attribute_name = 0
__hidden_attribute_name = 0
Here is a representative style guide for grammar.
Limit one line to 79 letters.
Write import at the top of the file and call in the order of built-in modules, third-party modules, and homemade modules.
Write the first factor of the instance method as self, and the first factor of the class method as cls.
Put a space back and forth of the assignment operator (=)
There is also a Python package that checks whether Python’s grammar complies with PEP 8, and Pylint and Flake8 are typical. There is also a Python package that automatically modifies the code style to the recommendations, and Black is a representative example. When using a Pycharm or Visual Studio Code editor, those packages can be conveniently used through the GUI.
Python style.
The grammar is strict because of its unique philosophy. Apart from whether it works well, there is even a style guide that recommends writing like this for readability. Python official documents recommend keeping the coding style suitable for Python, which is PEP 8.
In other languages, brackets are usually used in block units, but in Python, indentation is used instead of brackets. Because of this indentation grammar, the official coding guide specified in PEP 8 strongly recommends putting four characters of Space instead of Tab characters in source code indentation. Tab characters have different widths of spaces according to settings of a user or system. The problem is that the Python interpreter processes one tap character as one blank character. If you process the indentation with a tap character, the code indentation will be broken with a high probability if you open it in an editor with a different tap setting, and the source code will be broken in a form that humans cannot fix (visual block but Python interpreter recognizes as another block). Of course, programmers cannot let go of the annoying act of hitting the space bar four times. The latest editors used by Python developers, such as PyCharm and VSCode, support the ability to automatically convert into four gaps and input them, so using these editors eliminates the need to bother four gaps.
In Python, no matter how you name a variable or class, it works well. However, PEP 8 also specifies the recommended style for this. When coding Python, it is recommended to follow this recommended style for readability. Below is a representative style guide for names.
MODULE_CONSTANT_NAME = 0
import module_name
class ClassName()
def function_name()
variable_name = 0
_protected_attribute_name = 0
__hidden_attribute_name = 0
Here is a representative style guide for grammar.
Limit one line to 79 letters.
Write import at the top of the file and call in the order of built-in modules, third-party modules, and homemade modules.
Write the first factor of the instance method as self, and the first factor of the class method as cls.
Put a space back and forth of the assignment operator (=)
There is also a Python package that checks whether Python’s grammar complies with PEP 8, and Pylint and Flake8 are typical. There is also a Python package that automatically modifies the code style to the recommendations, and Black is a representative example. When using a Pycharm or Visual Studio Code editor, those packages can be conveniently used through the GUI.
Repeatable object.
One of the biggest features of Python. Python provides a powerful function of repeatable objects. This object can repeat sets, strings, lists, tuples, dictionaries, and even functions, which can be used in the for syntax. The list and tuple can be said to be a little more comfortable, but it is a great advantage to be able to repeat the value of the function. For example, when there is an f(n) function that calculates a multiple of n,
It is also possible to make a function such as. Even after calling the function, the local variable remains until the function is completely finished, and the local variable is deleted only after the function is finished. Therefore, each time a function is called, the value of x increases. The repeatable object created in this way may call values in order using grammar such as a _next function, a next object function, or a for…in object. In particular, in the case of generators, it is advantageous in terms of memory management because it is not made in advance, but because it is a method of creating and returning a new return value when called.